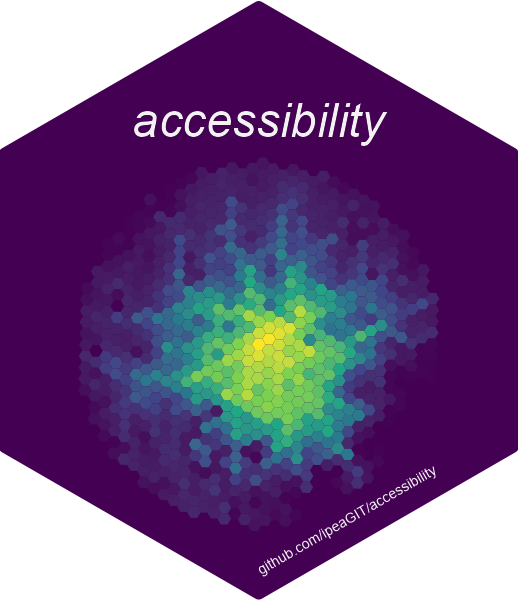
Minimum travel cost to closest N number of opportunities
Source:R/cost_to_closest.R
cost_to_closest.Rd
Calculates the minimum travel cost to the closest N number of opportunities.
This function is generic over any kind of numeric travel cost, such as distance, time and money.
Usage
cost_to_closest(
travel_matrix,
land_use_data,
opportunity,
travel_cost,
n = 1,
group_by = character(0),
active = TRUE,
fill_missing_ids = TRUE
)
Arguments
- travel_matrix
A data frame. The travel matrix describing the costs (i.e. travel time, distance, monetary cost, etc.) between the origins and destinations in the study area. Must contain the columns
from_id
,to_id
and any others specified intravel_cost
.- land_use_data
A data frame. The distribution of opportunities within the study area cells. Must contain the columns
id
and any others specified inopportunity
.- opportunity
A string. The name of the column in
land_use_data
with the number of opportunities/resources/services to be considered when calculating accessibility levels.- travel_cost
A string. The name of the column in
travel_matrix
with the travel cost between origins and destinations.- n
A
numeric
vector. The minimum number of opportunities that should be considered. Defaults to 1. If more than one value is provided, the output includes an extra column specifying the number of opportunities that the minimum travel cost refers to.- group_by
A
character
vector. When notcharacter(0)
(the default), indicates thetravel_matrix
columns that should be used to group the accessibility estimates by. For example, iftravel_matrix
includes a departure time column, that specifies the departure time of each entry in the data frame, passing"departure_time"
to this parameter results in accessibility estimates grouped by origin and by departure time.- active
A logical. Whether to calculate active accessibility (the of opportunities that can be reached from a given origin, the default) or passive accessibility (by how many people each destination can be reached).
- fill_missing_ids
A
logical
. Calculating minimum travel cost to closest N number of opportunities may result in missing ids in the output if they cannot reach the specified amount of opportunities across all destinations they can reach. For example, estimating the minimum travel time that an origin that can only reach 4 opportunities takes to reach 5 opportunities resulting in such origin not being included in the output. WhenTRUE
(the default), the function identifies which ids would be left out from the output and fill their respective minimum travel costs withInf
, which incurs in a performance penalty.
Value
A data frame containing the accessibility estimates for each
origin/destination (depending if active
is TRUE
or FALSE
) in the
travel matrix.
Examples
if (FALSE) { # identical(tolower(Sys.getenv("NOT_CRAN")), "true")
data_dir <- system.file("extdata", package = "accessibility")
travel_matrix <- readRDS(file.path(data_dir, "travel_matrix.rds"))
land_use_data <- readRDS(file.path(data_dir, "land_use_data.rds"))
df <- cost_to_closest(
travel_matrix,
land_use_data,
n = 1,
opportunity = "schools",
travel_cost = "travel_time"
)
head(df)
df <- cost_to_closest(
travel_matrix,
land_use_data,
n = c(1, 2),
opportunity = "schools",
travel_cost = "travel_time"
)
head(df)
}