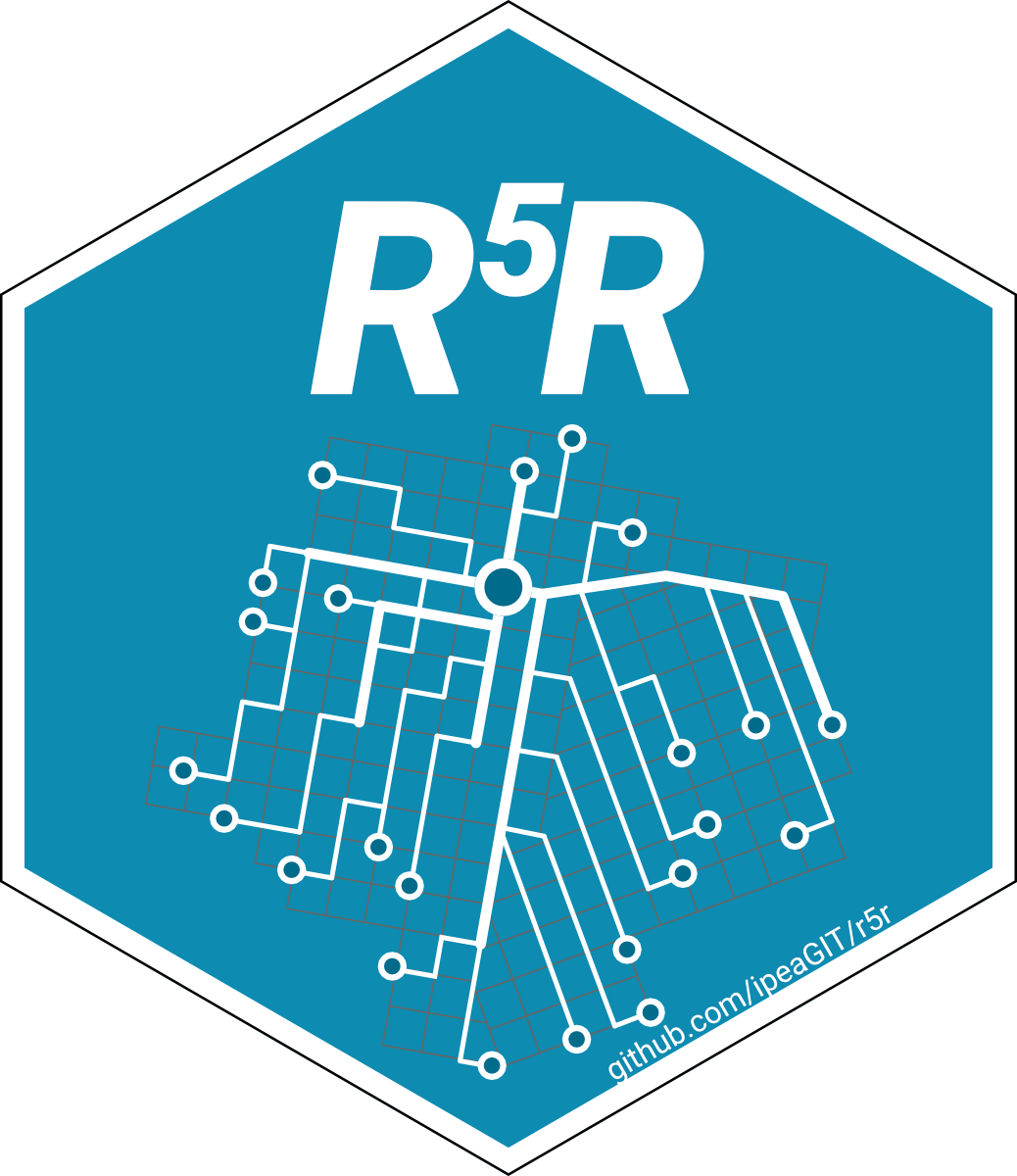
Calculate minute-by-minute travel times between origin destination pairs
Source:R/expanded_travel_time_matrix.R
expanded_travel_time_matrix.Rd
Detailed computation of travel time estimates between one or multiple origin destination pairs. Results show the travel time of the fastest route alternative departing each minute within a specified time window. Please note this function can be very memory intensive for large data sets and time windows.
Usage
expanded_travel_time_matrix(
r5r_core,
origins,
destinations,
mode = "WALK",
mode_egress = "WALK",
departure_datetime = Sys.time(),
time_window = 10L,
breakdown = FALSE,
max_walk_time = Inf,
max_bike_time = Inf,
max_car_time = Inf,
max_trip_duration = 120L,
walk_speed = 3.6,
bike_speed = 12,
max_rides = 3,
max_lts = 2,
draws_per_minute = 5L,
n_threads = Inf,
verbose = FALSE,
progress = FALSE,
output_dir = NULL
)
Arguments
- r5r_core
An object to connect with the R5 routing engine, created with
setup_r5()
.- origins, destinations
Either a
POINT sf
object with WGS84 CRS, or adata.frame
containing the columnsid
,lon
andlat
.- mode
A character vector. The transport modes allowed for access, transfer and vehicle legs of the trips. Defaults to
WALK
. Please see details for other options.- mode_egress
A character vector. The transport mode used after egress from the last public transport. It can be either
WALK
,BICYCLE
orCAR
. Defaults toWALK
. Ignored when public transport is not used.- departure_datetime
A POSIXct object. Please note that the departure time only influences public transport legs. When working with public transport networks, please check the
calendar.txt
within your GTFS feeds for valid dates. Please see details for further information on how datetimes are parsed.- time_window
An integer. The time window in minutes for which
r5r
will calculate multiple travel time matrices departing each minute. Defaults to 10 minutes. The function returns the result based on median travel times. Please read the time window vignette for more details on its usagevignette("time_window", package = "r5r")
- breakdown
A logical. Whether to include detailed information about each trip in the output. If
FALSE
(the default), the output lists the total time between each origin-destination pair and the routes used to complete the trip for each minute of the specified time window. IfTRUE
, the output includes the total access, waiting, in-vehicle and transfer time of each trip. Please note that setting this parameter toTRUE
makes the function significantly slower.- max_walk_time
An integer. The maximum walking time (in minutes) to access and egress the transit network, to make transfers within the network or to complete walk-only trips. Defaults to no restrictions (numeric value of
Inf
), as long asmax_trip_duration
is respected. When routing transit trips, the max time is considered separately for each leg (e.g. if you setmax_walk_time
to 15, you could get trips with an up to 15 minutes walk leg to reach transit and another up to 15 minutes walk leg to reach the destination after leaving transit. In walk-only trips, whenevermax_walk_time
differs frommax_trip_duration
, the lowest value is considered.- max_bike_time
An integer. The maximum cycling time (in minutes) to access and egress the transit network, to make transfers within the network or to complete bicycle-only trips. Defaults to no restrictions (numeric value of
Inf
), as long asmax_trip_duration
is respected. When routing transit trips, the max time is considered separately for each leg (e.g. if you setmax_bike_time
to 15, you could get trips with an up to 15 minutes cycle leg to reach transit and another up to 15 minutes cycle leg to reach the destination after leaving transit. In bicycle-only trips, whenevermax_bike_time
differs frommax_trip_duration
, the lowest value is considered.- max_car_time
An integer. The maximum driving time (in minutes) to access and egress the transit network. Defaults to no restrictions, as long as
max_trip_duration
is respected. The max time is considered separately for each leg (e.g. if you setmax_car_time
to 15 minutes, you could potentially drive up to 15 minutes to reach transit, and up to another 15 minutes to reach the destination after leaving transit). Defaults toInf
, no limit.- max_trip_duration
An integer. The maximum trip duration in minutes. Defaults to 120 minutes (2 hours).
- walk_speed
A numeric. Average walk speed in km/h. Defaults to 3.6 km/h.
- bike_speed
A numeric. Average cycling speed in km/h. Defaults to 12 km/h.
- max_rides
An integer. The maximum number of public transport rides allowed in the same trip. Defaults to 3.
- max_lts
An integer between 1 and 4. The maximum level of traffic stress that cyclists will tolerate. A value of 1 means cyclists will only travel through the quietest streets, while a value of 4 indicates cyclists can travel through any road. Defaults to 2. Please see details for more information.
- draws_per_minute
An integer. The number of Monte Carlo draws to perform per time window minute when calculating travel time matrices and when estimating accessibility. Defaults to 5. This would mean 300 draws in a 60-minute time window, for example. This parameter only affects the results when the GTFS feeds contain a
frequencies.txt
table. If the GTFS feed does not have a frequency table, r5r still allows for multiple runs over the settime_window
but in a deterministic way.- n_threads
An integer. The number of threads to use when running the router in parallel. Defaults to use all available threads (Inf).
- verbose
A logical. Whether to show
R5
informative messages when running the function. Defaults toFALSE
(please note that in such caseR5
error messages are still shown). Settingverbose
toTRUE
shows detailed output, which can be useful for debugging issues not caught byr5r
.- progress
A logical. Whether to show a progress counter when running the router. Defaults to
FALSE
. Only works whenverbose
is set toFALSE
, so the progress counter does not interfere withR5
's output messages. Settingprogress
toTRUE
may impose a small penalty for computation efficiency, because the progress counter must be synchronized among all active threads.- output_dir
Either
NULL
or a path to an existing directory. When notNULL
(the default), the function will write one.csv
file with the results for each origin in the specified directory. In such case, the function returns the path specified in this parameter. This parameter is particularly useful when running on memory-constrained settings because writing the results directly to disk preventsr5r
from loading them to RAM memory.
Value
A data.table
with travel time estimates (in minutes) and the
routes used in each trip between origin and destination pairs, for each
minute of the specified time window. Each set of origin, destination and
departure minute can appear up to N times, where N is the number of Monte
Carlo draws specified in the function arguments (please note that this
only applies when the GTFS feeds that describe the transit network include
a frequencies table, otherwise only a single draw is performed). A pair is
completely absent from the final output if no trips could be completed in
any of the minutes of the time window. If for a single pair trips could be
completed in some of the minutes of the time window, but not for all of
them, the minutes in which trips couldn't be completed will have NA
travel time and routes used. If output_dir
is not NULL
, the function
returns the path specified in that parameter, in which the .csv
files
containing the results are saved.
Transport modes
R5
allows for multiple combinations of transport modes. The options
include:
Transit modes:
TRAM
,SUBWAY
,RAIL
,BUS
,FERRY
,CABLE_CAR
,GONDOLA
,FUNICULAR
. The optionTRANSIT
automatically considers all public transport modes available.Non transit modes:
WALK
,BICYCLE
,CAR
,BICYCLE_RENT
,CAR_PARK
.
Level of Traffic Stress (LTS)
When cycling is enabled in R5
(by passing the value BIKE
to either
mode
or mode_egress
), setting max_lts
will allow cycling only on
streets with a given level of danger/stress. Setting max_lts
to 1, for
example, will allow cycling only on separated bicycle infrastructure or
low-traffic streets and routing will revert to walking when traversing any
links with LTS exceeding 1. Setting max_lts
to 3 will allow cycling on
links with LTS 1, 2 or 3. Routing also reverts to walking if the street
segment is tagged as non-bikable in OSM (e.g. a staircase), independently of
the specified max LTS.
The default methodology for assigning LTS values to network edges is based on commonly tagged attributes of OSM ways. See more info about LTS in the original documentation of R5 from Conveyal at https://docs.conveyal.com/learn-more/traffic-stress. In summary:
LTS 1: Tolerable for children. This includes low-speed, low-volume streets, as well as those with separated bicycle facilities (such as parking-protected lanes or cycle tracks).
LTS 2: Tolerable for the mainstream adult population. This includes streets where cyclists have dedicated lanes and only have to interact with traffic at formal crossing.
LTS 3: Tolerable for "enthused and confident" cyclists. This includes streets which may involve close proximity to moderate- or high-speed vehicular traffic.
LTS 4: Tolerable only for "strong and fearless" cyclists. This includes streets where cyclists are required to mix with moderate- to high-speed vehicular traffic.
For advanced users, you can provide custom LTS values by adding a tag <key = "lts">
to the osm.pbf
file.
Datetime parsing
r5r
ignores the timezone attribute of datetime objects when parsing dates
and times, using the study area's timezone instead. For example, let's say
you are running some calculations using Rio de Janeiro, Brazil, as your study
area. The datetime as.POSIXct("13-05-2019 14:00:00", format = "%d-%m-%Y %H:%M:%S")
will be parsed as May 13th, 2019, 14:00h in
Rio's local time, as expected. But as.POSIXct("13-05-2019 14:00:00", format = "%d-%m-%Y %H:%M:%S", tz = "Europe/Paris")
will also be parsed as
the exact same date and time in Rio's local time, perhaps surprisingly,
ignoring the timezone attribute.
Routing algorithm
The travel_time_matrix()
, expanded_travel_time_matrix()
and
accessibility()
functions use an R5
-specific extension to the RAPTOR
routing algorithm (see Conway et al., 2017). This RAPTOR extension uses a
systematic sample of one departure per minute over the time window set by the
user in the 'time_window' parameter. A detailed description of base RAPTOR
can be found in Delling et al (2015). However, whenever the user includes
transit fares inputs to these functions, they automatically switch to use an
R5
-specific extension to the McRAPTOR routing algorithm.
Conway, M. W., Byrd, A., & van der Linden, M. (2017). Evidence-based transit and land use sketch planning using interactive accessibility methods on combined schedule and headway-based networks. Transportation Research Record, 2653(1), 45-53. doi:10.3141/2653-06
Delling, D., Pajor, T., & Werneck, R. F. (2015). Round-based public transit routing. Transportation Science, 49(3), 591-604. doi:10.1287/trsc.2014.0534
See also
Other routing:
detailed_itineraries()
,
pareto_frontier()
,
travel_time_matrix()
Examples
library(r5r)
# build transport network
data_path <- system.file("extdata/poa", package = "r5r")
r5r_core <- setup_r5(data_path)
#>
#> Using cached network.dat from /home/runner/work/_temp/Library/r5r/extdata/poa/network.dat
# load origin/destination points
points <- read.csv(file.path(data_path, "poa_points_of_interest.csv"))
departure_datetime <- as.POSIXct(
"13-05-2019 14:00:00",
format = "%d-%m-%Y %H:%M:%S"
)
# by default only returns the total time between each pair in each minute of
# the specified time window
ettm <- expanded_travel_time_matrix(
r5r_core,
origins = points,
destinations = points,
mode = c("WALK", "TRANSIT"),
time_window = 20,
departure_datetime = departure_datetime,
max_trip_duration = 60
)
head(ettm)
#> from_id to_id departure_time draw_number routes total_time
#> <char> <char> <char> <int> <char> <num>
#> 1: public_market public_market 14:00:00 1 [WALK] 0
#> 2: public_market public_market 14:01:00 1 [WALK] 0
#> 3: public_market public_market 14:02:00 1 [WALK] 0
#> 4: public_market public_market 14:03:00 1 [WALK] 0
#> 5: public_market public_market 14:04:00 1 [WALK] 0
#> 6: public_market public_market 14:05:00 1 [WALK] 0
# when breakdown = TRUE the output contains much more information
ettm <- expanded_travel_time_matrix(
r5r_core,
origins = points,
destinations = points,
mode = c("WALK", "TRANSIT"),
time_window = 20,
departure_datetime = departure_datetime,
max_trip_duration = 60,
breakdown = TRUE
)
head(ettm)
#> from_id to_id departure_time draw_number access_time wait_time
#> <char> <char> <char> <int> <num> <num>
#> 1: public_market public_market 14:00:00 1 0 0
#> 2: public_market public_market 14:01:00 1 0 0
#> 3: public_market public_market 14:02:00 1 0 0
#> 4: public_market public_market 14:03:00 1 0 0
#> 5: public_market public_market 14:04:00 1 0 0
#> 6: public_market public_market 14:05:00 1 0 0
#> ride_time transfer_time egress_time routes n_rides total_time
#> <num> <num> <num> <char> <int> <num>
#> 1: 0 0 0 [WALK] 0 0
#> 2: 0 0 0 [WALK] 0 0
#> 3: 0 0 0 [WALK] 0 0
#> 4: 0 0 0 [WALK] 0 0
#> 5: 0 0 0 [WALK] 0 0
#> 6: 0 0 0 [WALK] 0 0
stop_r5(r5r_core)
#> r5r_core has been successfully stopped.